
HackerRank Lisa’s Workbook Problem Solution
In this post, we will solve HackerRank Lisa’s Workbook Problem Solution.
Lisa just got a new math workbook. A workbook contains exercise problems, grouped into chapters. Lisa believes a problem to be special if its index (within a chapter) is the same as the page number where it’s located. The format of Lisa’s book is as follows:
- There are n chapters in Lisa’s workbook, numbered from 1 to n.
The ¿th chapter has arr[i] problems, numbered from 1 to arr[i]. - Each page can hold up to k problems. Only a chapter’s last page of exercises may contain fewer than k problems.
- Each new chapter starts on a new page, so a page will never contain problems from more than one chapter.
- The page number indexing starts at 1.
Given the details for Lisa’s workbook, can you count its number of special problems?
Example
arr = [4,2]
k = 3
Lisa’s workbook contains arr[1] = 4 problems for chapter 1, and arr[2] = 2 problems for chapter 2. Each page can hold k = 3 problems.
The first page will hold 3 problems for chapter 1. Problem 1 is on page 1, so it is special. Page 2 contains only Chapter 1, Problem 4, so no special problem is on page 2. Chapter 2 problems start on page 3 and there are 2 problems. Since there is no problem 3 on page 3, there is no special problem on that page either. There is 1 special problem in her workbook.
Note: See the diagram in the Explanation section for more details.
Function Description
Complete the workbook function in the editor below.
workbook has the following parameter(s):
- int n: the number of chapters
- int k: the maximum number of problems per page
- int arr[n]: the number of problems in each chapter
Returns
– int: the number of special problems in the workbook
Input Format
The first line contains two integers n and k, the number of chapters and the maximum
number of problems per page.
The second line contains n space-separated integers arr[i] where arr[i] denotes the number of problems in the ¿th chapter.
Sample Input
STDIN Function ----- -------- 5 3 n = 5, k = 3 4 2 6 1 10 arr = [4, 2, 6, 1, 10]
Sample Output
4
Explanation
The diagram below depicts Lisa’s workbook with n = 5 chapters and a maximum of k= 3 problems per page. Special problems are outlined in red, and page numbers are in yellow squares.
There are 4 special problems and thus we print the number 4 on a new line.
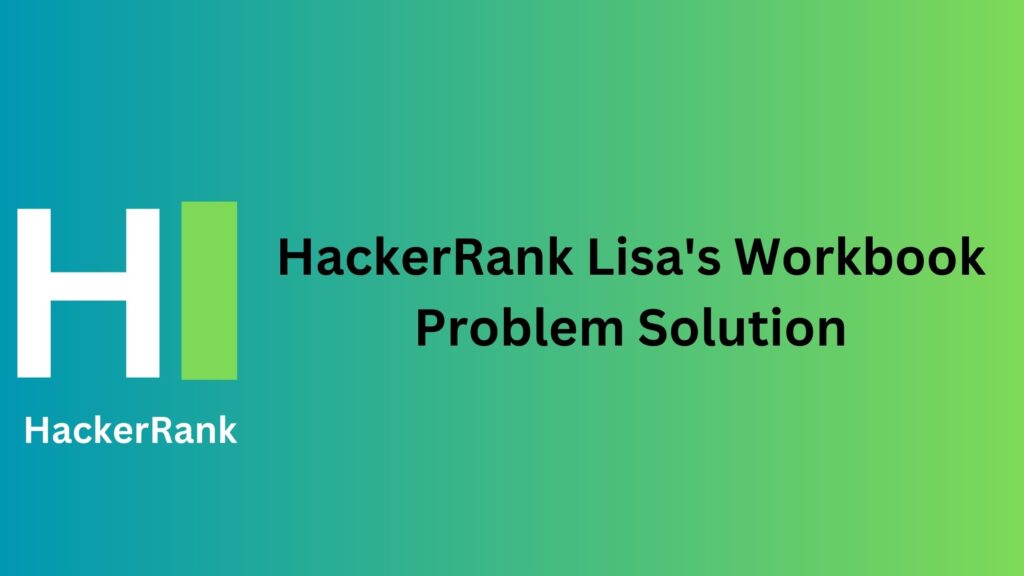
Lisa’s Workbook C Solution
#include <stdio.h>
#include <math.h>
int main()
{
//printf("dfgh");
int n, k, i, u, j, i_p, l_p, ttl_p, m_n, count;
double m;
scanf("%d %d", &n, &k);
int pr[n];
for(i=0; i<n; i++){
scanf("%d", &pr[i]);
}
count = 0;
l_p = 0;
for(i=0; i<n; i++){
m = (pr[i] * 1.00)/k;
ttl_p = ceil(m);
i_p = l_p + 1;
l_p = i_p + ttl_p - 1;
m_n = 0;
for(j=i_p; j<=l_p; j++){
for(u=0; u<k; u++){
m_n++;
if(j==m_n) count++;
if(m_n==pr[i]) break;
}
}
}
printf("%d\n", count);
return 0;
}
Lisa’s Workbook C++ Solution
#include<iostream>
#include<algorithm>
#include<vector>
#include<map>
#include<string>
#include<sstream>
#include<cmath>
#include<math.h>
using namespace std;
int main()
{
int n, k, t;
int p = 1;
int r = 0;
cin >> n >> k;
for (int i = 1; i <= n; i++)
{
cin >> t;
for (int x = 1; x <= t; x++)
{
if (p == x)
r++;
if (x % k == 0)
p++;
}
if (t % k != 0)
p++;
}
cout << r << endl;
return 0;
}
Lisa’s Workbook C Sharp Solution
using System;
using System.Collections.Generic;
using System.IO;
class Solution
{
static void Main(String[] args)
{
int[] n = Array.ConvertAll(Console.ReadLine().Split(' '), int.Parse);
int currentPage = 1;
int numSpecial = 0;
int[] data = Array.ConvertAll(Console.ReadLine().Split(' '), int.Parse);
for (int i = 0; i < n[0]; i++)
{
int question = 1;
while (question <= data[i])
{
if (question == currentPage)
{
numSpecial++;
}
if (question % n[1] == 0 && question != data[i])
currentPage++;
question++;
}
currentPage++;
}
Console.WriteLine(numSpecial);
}
}
Lisa’s Workbook Java Solution
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
class Result {
/*
* Complete the 'workbook' function below.
*
* The function is expected to return an INTEGER.
* The function accepts following parameters:
* 1. INTEGER n
* 2. INTEGER k
* 3. INTEGER_ARRAY arr
*/
public static int workbook(int n, int k, List<Integer> arr) {
int page = 1;
int numSpecials = 0;
for (int i = 0; i < n; ++i) {
int numProblems = arr.get(i);
int problem = 1;
while (numProblems > 0) {
int pageProblems = numProblems;
pageProblems -= k;
if (pageProblems > 0) {
pageProblems = k;
} else {
pageProblems += k;
}
while (pageProblems > 0) {
if (page == problem) {
++numSpecials;
}
++problem;
--pageProblems;
}
numProblems -= k;
++page;
}
}
return numSpecials;
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
String[] firstMultipleInput = bufferedReader.readLine().replaceAll("\\s+$", "").split(" ");
int n = Integer.parseInt(firstMultipleInput[0]);
int k = Integer.parseInt(firstMultipleInput[1]);
List<Integer> arr = Stream.of(bufferedReader.readLine().replaceAll("\\s+$", "").split(" "))
.map(Integer::parseInt)
.collect(toList());
int result = Result.workbook(n, k, arr);
bufferedWriter.write(String.valueOf(result));
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
Lisa’s Workbook Javascript Solution
function processData(input) {
//Enter your code here
input = input.split('\n');
var chapters = parseInt(input[0].split(' ')[0], 10);
var perPage = parseInt(input[0].split(' ')[1], 10);
var perChapter = input[1].split(' ');
for(var i=0; i<chapters; i++) perChapter[i] = parseInt(perChapter[i], 10);
var result = 0;
var page = 1;
for(var i=1; i<=chapters; i++) {
for(var j=1; j<=perChapter[i-1]; j++) {
if(j>1 && ((j-1) % perPage === 0)) page++;
if(j===page) result++;
}
page++;
}
console.log(result);
}
process.stdin.resume();
process.stdin.setEncoding("ascii");
_input = "";
process.stdin.on("data", function (input) {
_input += input;
});
process.stdin.on("end", function () {
processData(_input);
});
Lisa’s Workbook Python Solution
from math import ceil
def get_split(x, k):
r = []
for i in range(ceil(x/k)):
r.append(tuple(range(i*k+1, min((i+1)*k, x)+1)))
return r
n, k = [int(x) for x in input().split()]
t = [int(x) for x in input().split()]
p = [ceil(x/k) for x in t]
r = [i for s in [get_split(x, k) for x in t] for i in s]
s = 0
for i in range(sum(p)):
if ((i+1) in r[i]):
s += 1
print(s)
Other Solutions
Good post and right to the point. I don’t know if this is really the best place to ask but do you guys have any ideea where to hire some professional writers?
Thx 🙂 Escape room lista
Very interesting topic, thank you for putting up.!