
HackerRank Mars Exploration Problem Solution
In this post, we will solve HackerRank Mars Exploration Problem Solution. A space explorer’s ship crashed on Mars! They send a series of SOS
messages to Earth for help.
Letters in some of the SOS
messages are altered by cosmic radiation during transmission. Given the signal received by Earth as a string, s, determine how many letters of the SOS
message have been changed by radiation.
Example
s = SOSTOT
The original message was SOSSOS
. Two of the message’s characters were changed in transit.
Function Description
Complete the marsExploration function in the editor below.
marsExploration has the following parameter(s):
- string s: the string as received on Earth
Returns
- int: the number of letters changed during transmission
Input Format
There is one line of input: a single string, s.
Sample Input 0
SOSSPSSQSSOR
Sample Output 0
3
Explanation 0
8 = SOSSPSSQSSOR, and signal length |s|= 12. They sent 4 sos messages (i.e.: 12/3 = 4).
Expected signal: SOSSOSSOSSOS Recieved signal: SOSSPSSQSSOR Difference: X X X
Sample Input 1
SOSSOT
Sample Output 1
1
Explanation 1
S = SOSSOT, and signal length |s = 6. They sent 2 sos messages (i.e.: 6/3 = 2).
Sample Input 2
SOSSOSSOS
Sample Output 2
0
Explanation 2
Since no character is altered, return 0.
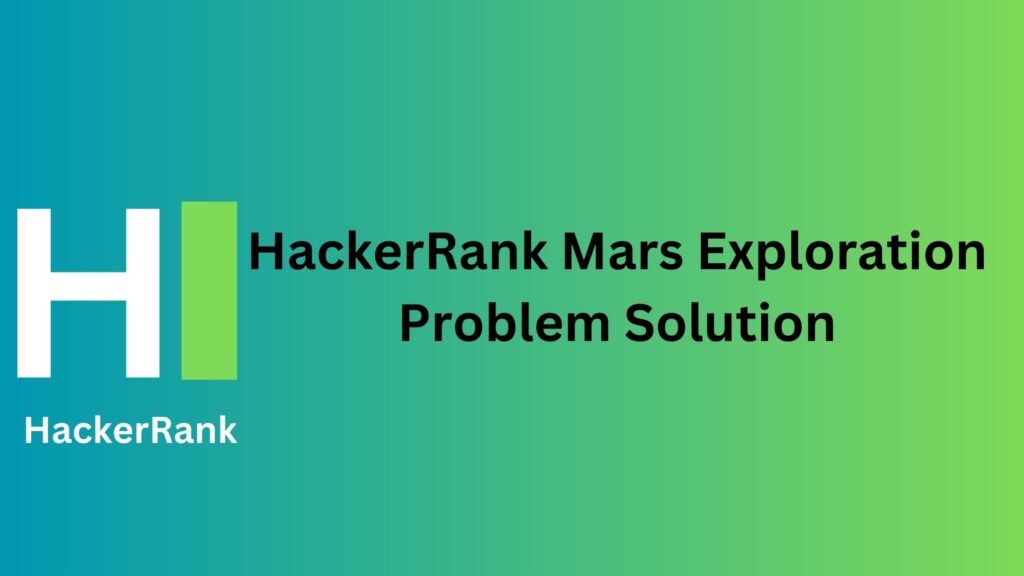
Mars Exploration C Solution
#include <math.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <assert.h>
#include <limits.h>
#include <stdbool.h>
int main(){
int p, n;
char* S = (char *)malloc(10240 * sizeof(char));
scanf("%s",S);
n = p = 0;
while (S[p] != '\0')
{
if (S[p++] != 'S') n++;
if (S[p++] != 'O') n++;
if (S[p++] != 'S') n++;
}
printf ("%d\n", n);
return 0;
}
Mars Exploration C++ Solution
#include <cmath>
#include <cstdio>
#include <vector>
#include <iostream>
#include <algorithm>
using namespace std;
int main() {
/* Enter your code here. Read input from STDIN. Print output to STDOUT */
auto f = [&](const std::string& s){
int cnt = 0;
std::string sos("SOS");
for(size_t i = 0; i < s.length(); ++i){
if(s[i] != sos[i%3]) cnt++;
}
return cnt;
};
std::string s;
std::cin >> s;
std::cout << f(s) << std::endl;
return 0;
}
Mars Exploration C Sharp Solution
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
class Solution {
static void Main(String[] args) {
string S = Console.ReadLine();
string t = "";
int count = 0;
for(int i = 0; i < S.Length; i+=3){
t = S.Substring(i,3);
if(t.Substring(0,1) != "S"){
count ++;
}
if(t.Substring(1,1) != "O"){
count ++;
}
if(t.Substring(2,1) != "S"){
count ++;
}
}
Console.WriteLine(count);
}
}
Mars Exploration Java Solution
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
class Result {
/*
* Complete the 'marsExploration' function below.
*
* The function is expected to return an INTEGER.
* The function accepts STRING s as parameter.
*/
public static int marsExploration(String s) {
int count = 0;
for(int i = 0;i<s.length()-2;i+=3) {
if(!s.substring(i, i+1).equals("S")) {
count++;
}
if(!s.substring(i+1, i+2).equals("O")) {
count++;
}
if(!s.substring(i+2, i+3).equals("S")) {
count++;
}
}
return count;
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
String s = bufferedReader.readLine();
int result = Result.marsExploration(s);
bufferedWriter.write(String.valueOf(result));
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
Mars Exploration JavaScript Solution
process.stdin.resume();
process.stdin.setEncoding('ascii');
var input_stdin = "";
var input_stdin_array = "";
var input_currentline = 0;
process.stdin.on('data', function (data) {
input_stdin += data;
});
process.stdin.on('end', function () {
input_stdin_array = input_stdin.split("\n");
main();
});
function readLine() {
return input_stdin_array[input_currentline++];
}
/////////////// ignore above this line ////////////////////
function main() {
var s = readLine();
console.log(unexpectedLetters(s));
}
function unexpectedLetters(s) {
var expected = 0;
for (var i = 0; i < s.length; i+= 3) {
expected += boolToInt(s[i] === 'S') +
boolToInt(s[i + 1] === 'O') +
boolToInt(s[i + 2] === 'S');
}
return s.length - expected;
}
function boolToInt(expr) {
return expr ? 1 : 0;
}
Mars Exploration Python Solution
#!/bin/python3
import sys
S = input().strip()
count = 0
for i in range(len(S)):
if i % 3 == 0 or i % 3 == 2:
if S[i] != 'S':
count += 1
elif i % 3 == 1 and S[i] != 'O':
count += 1
print(count)
Other Solutions