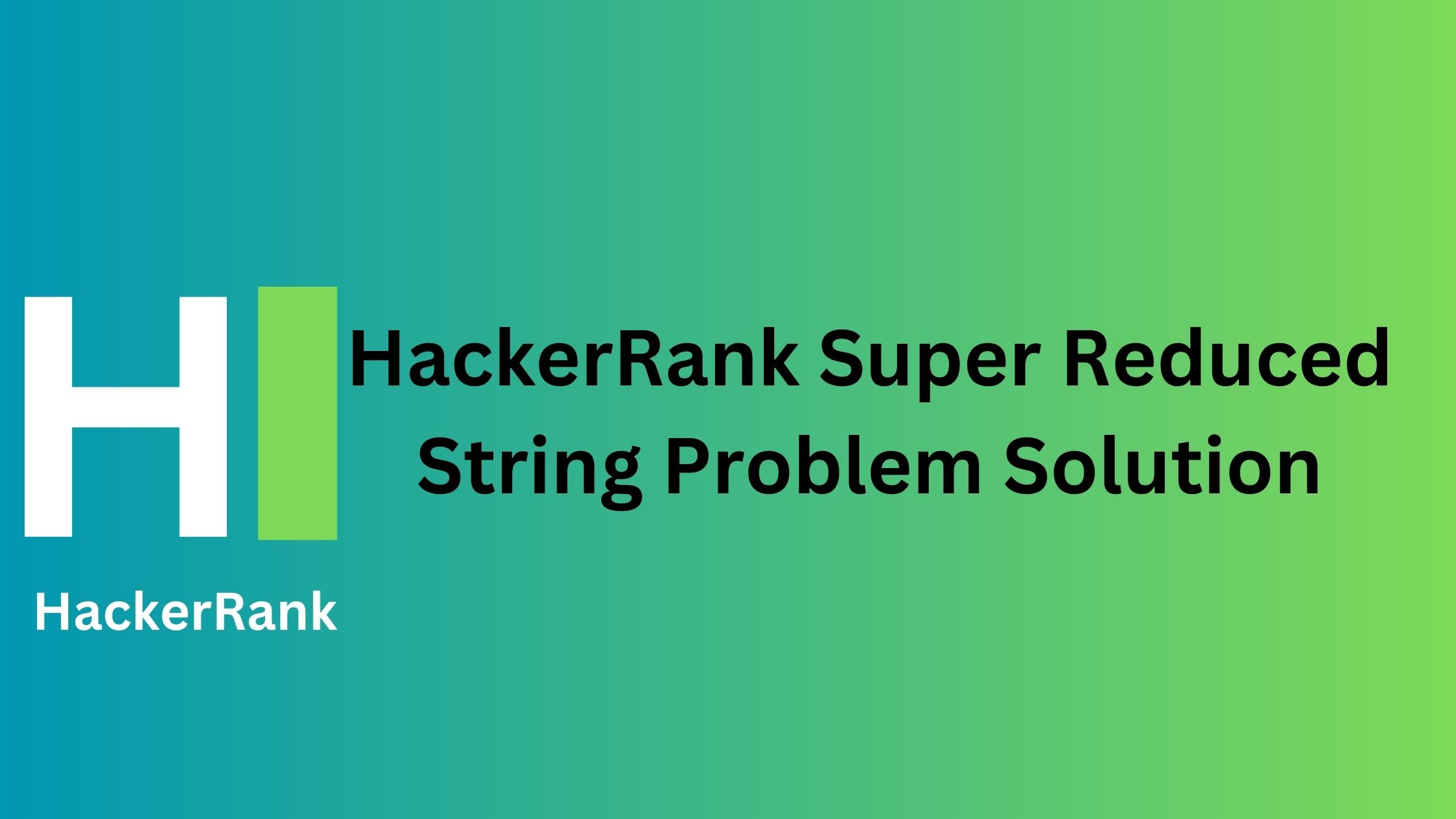
HackerRank Super Reduced String Solution
In this post, we will solve HackerRank Super Reduced String Problem Solution.
Reduce a string of lowercase characters in range ascii [‘a’..’z’] by doing a series of operations. In each operation, select a pair of adjacent letters that match, and delete them. Delete as many characters as possible using this method and return the resulting string. If the final string is empty, return Empty String
Example
s = ‘aab’
aab shortens to b in one operation: remove the adjacent a characters.
s = ‘abba’
Remove the two ‘b’ characters leaving ‘aa’. Remove the two ‘a’ characters to leave “. Return ‘Empty String’.
Function Description
Complete the superReducedString function in the editor below.
superReducedString has the following parameter(s):
- string s: a string to reduce
Returns
- string: the reduced string or
Empty String
Input Format
A single string, s
Sample Input 0
aaabccddd
Sample Output 0
abd
Explanation 0
Perform the following sequence of operations to get the final string:
aaabccddd → abccddd → abddd → abd
Sample Input 1
aa
Sample Output 1
Empty String
Explanation 1
aa → Empty String
Sample Input 2
baab
Sample Output 2
Empty String
Explanation 2
baab → bb → Empty String

Super Reduced String C Solution
#include <stdio.h>
#include <string.h>
#include <math.h>
#include <stdlib.h>
int main() {
char s[103];
scanf("%s", s);
int len, ll;
do {
len = strlen(s);
// printf("%d\n", len);
for (char *ss=s; *ss; ss++) {
if (*ss == ss[1]) {
// printf("%s ", s );
strcpy(ss, ss+2);
// printf("%s\n", s );
} else {
// printf("\n");
}
}
ll = strlen(s);
// printf("%d\n", ll);
} while (len != ll);
if (s[0]==0) {
printf("Empty String\n");
} else {
printf("%s\n", s);
}
/* Enter your code here. Read input from STDIN. Print output to STDOUT */
return 0;
}
Super Reduced String C++ Solution
#include<bits/stdc++.h>
using namespace std;
#define pb push_back
#define all(c) (c).begin(), (c).end()
#define mp make_pair
string s, tmp = "";
int c = 0;
char str;
bool vis[105];
int main(){
ios_base :: sync_with_stdio(0);
cin >> s;
while(1){
if(c++ == 10000) break;
for(int i = 1; i < (int)s.size(); i++){
if((!vis[i]) && (!vis[i-1])){
if(s[i] == s[i-1]) vis[i] = 1, vis[i-1] = 1;
}
}
for(int i = 0; i < (int)s.size(); i++){
if(!vis[i]) tmp += s[i];
}
s = tmp; tmp = ""; memset(vis, 0, sizeof vis);
}
if(s == "") cout << "Empty String" << '\n';
else cout << s << '\n';
return 0;
}
Super Reduced String C Sharp Solution
using System;
using System.Collections.Generic;
using System.IO;
class Solution {
static void Main(String[] args)
{
string inputStr = Console.ReadLine();
while(true)
{
bool found = false;
for(int i = 0 ; i < inputStr.Length - 1; i++)
{
if( inputStr[i]==inputStr[i+1] )
{
inputStr = inputStr.Remove(i,2);
found = true;
continue;
}
}
if(!found)
break;
}
if(inputStr.Length == 0)
Console.WriteLine("Empty String");
else
Console.WriteLine(inputStr);
}
}
Super Reduced String Java Solution
import java.io.*;
import java.math.*;
import java.security.*;
import java.text.*;
import java.util.*;
import java.util.concurrent.*;
import java.util.function.*;
import java.util.regex.*;
import java.util.stream.*;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
class Result {
/*
* Complete the 'superReducedString' function below.
*
* The function is expected to return a STRING.
* The function accepts STRING s as parameter.
*/
public static String superReducedString(String s) {
if(s.length()==1){
return s;
}
char[] chars = s.toCharArray();
LinkedList<Character> charStack = new LinkedList<>();
for (int i=0;i<chars.length;i++){
Character last = charStack.peekLast();
if(last != null && last.equals(chars[i])){
charStack.removeLast();
}else{
charStack.addLast(chars[i]);
}
}
// print charStackContent
if(charStack.isEmpty()){
return "Empty String";
}
return charStack.stream().map(c->c.toString()).collect(Collectors.joining());
}
}
public class Solution {
public static void main(String[] args) throws IOException {
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(System.getenv("OUTPUT_PATH")));
String s = bufferedReader.readLine();
String result = Result.superReducedString(s);
bufferedWriter.write(result);
bufferedWriter.newLine();
bufferedReader.close();
bufferedWriter.close();
}
}
Super Reduced String JavaScript Solution
function processData(input) {
var s = input.concat();
for(i=0; i < s.length-1; )
{
if(s[i] === s[i+1])
{
s = s.slice(0, i) + s.slice(i+2);
i = 0;
}
else
{
i++;
}
}
console.log(s || "Empty String");
}
process.stdin.resume();
process.stdin.setEncoding("ascii");
_input = "";
process.stdin.on("data", function (input) {
_input += input;
});
process.stdin.on("end", function () {
processData(_input);
});
Super Reduced String Python Solution
s = input()
st = []
c = 0
cur = s[0]
for i in s:
if i == cur:
c += 1
else:
if c%2 != 0:
if st:
if st[-1] == cur:
st.pop(-1)
else:
st.append(cur)
else:
st.append(cur)
cur = i
c = 1
if c%2 != 0:
if st:
if st[-1] == cur:
st.pop(-1)
else:
st.append(cur)
else:
st.append(cur)
s = ''.join(st)
if not s:
s = "Empty String"
print(s)
Other Solutions